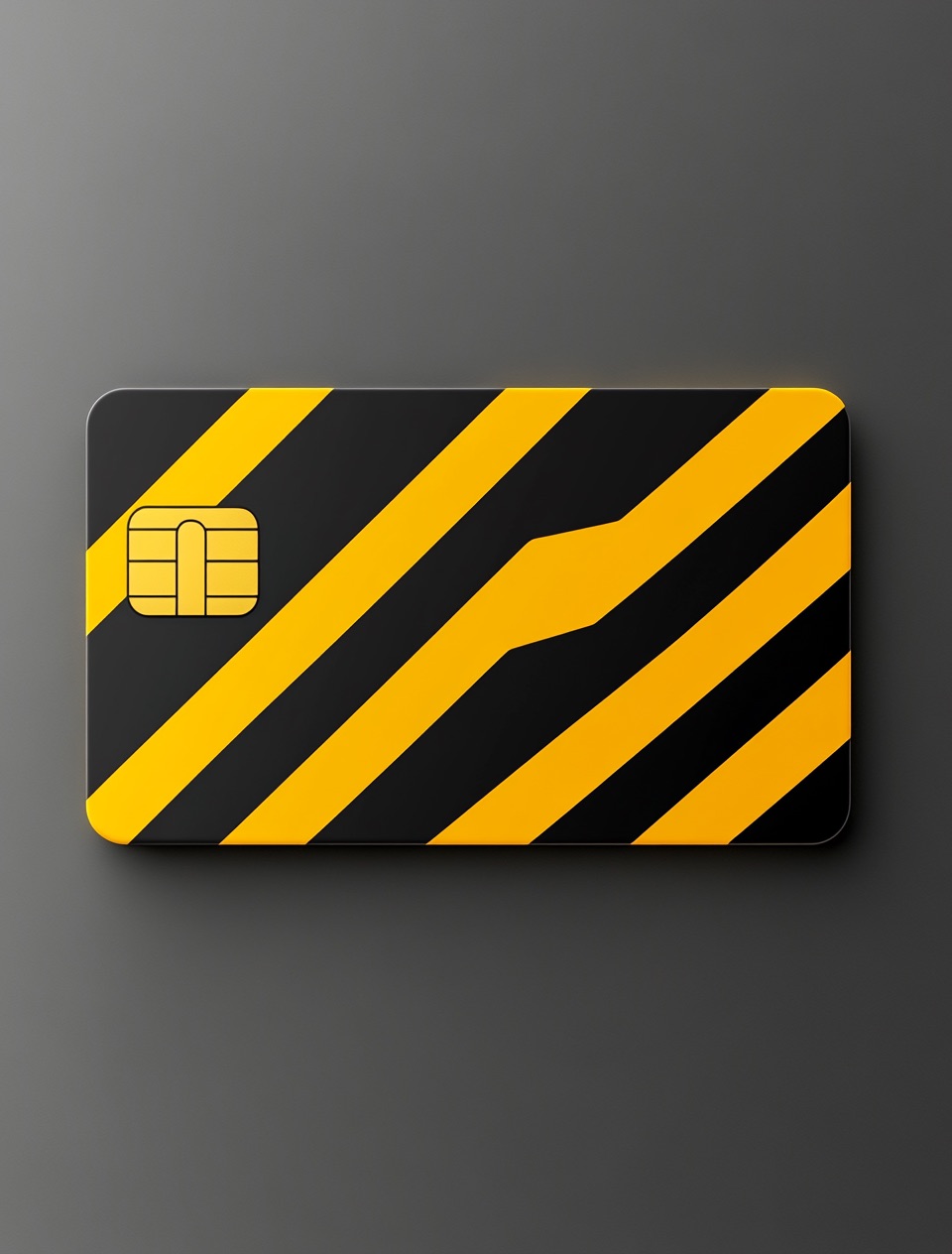
Understanding BIN Numbers: A Developer's Guide
What is a BIN/IIN Number?
Bank Identification Numbers (BIN) or Issuer Identification Numbers (IIN) are the first 6-8 digits of a payment card number that identify the card-issuing institution. These numbers are crucial for payment processing, fraud detection, and routing transactions.
BIN Number Structure
Standard Format Breakdown
Position | Length | Purpose | Example |
---|---|---|---|
1 | 1 digit | Major Industry Identifier (MII) | 4 (Banking) |
2-6 | 5 digits | Issuing Institution | 12345 |
7-8 | 2 digits | Extended BIN (optional) | 67 |
Major Industry Identifier (MII) Values
MII Digit | Industry |
---|---|
0 | ISO/TC 68 Assignment |
1 | Airlines |
2 | Airlines/Financial |
3 | Travel/Entertainment |
4 | Banking/Financial |
5 | Banking/Financial |
6 | Merchandising |
7 | Petroleum/Other |
8 | Healthcare/Telecommunications |
9 | National Assignment |
Common BIN Ranges by Card Network
Primary BIN Ranges
bin_ranges = {
'Visa': ['4'],
'Mastercard': ['51-55', '2221-2720'],
'American Express': ['34', '37'],
'Discover': ['6011', '644-649', '65'],
'JCB': ['3528-3589'],
'UnionPay': ['62']
}
BIN Lookup Implementation
def validate_bin(card_number):
bin_number = card_number[:6]
# Basic validation
if not bin_number.isdigit():
return False
# Network identification
if card_number.startswith('4'):
network = 'Visa'
elif any(card_number.startswith(str(i)) for i in range(51, 56)):
network = 'Mastercard'
elif card_number.startswith(('34', '37')):
network = 'American Express'
else:
network = 'Unknown'
return {
'bin': bin_number,
'network': network,
'length': len(card_number),
'valid': len(card_number) in [13, 15, 16, 19]
}
BIN Data Properties
Standard BIN Response Fields
Field | Description | Example |
---|---|---|
scheme | Card Network | "visa" |
country | Issuing Country | "US" |
type | Card Type | "credit" |
category | Card Category | "platinum" |
issuer | Issuing Bank | "CHASE" |
length | Card Number Length | 16 |
BIN-Based Validation Rules
Card Number Length by Network
Network | Valid Lengths |
---|---|
Visa | 13, 16, 19 |
Mastercard | 16 |
American Express | 15 |
Discover | 16, 19 |
UnionPay | 16-19 |
Integration Example
const binValidator = {
checkBIN: function(cardNumber) {
const bin = cardNumber.substring(0, 6);
const length = cardNumber.length;
// Basic format check
if (!/^\d{6}/.test(bin)) {
return {
valid: false,
error: 'Invalid BIN format'
};
}
// Network detection
const network = this.detectNetwork(bin);
// Length validation
const isValidLength = this.validateLength(length, network);
return {
valid: isValidLength,
network: network,
bin: bin,
cardLength: length
};
},
detectNetwork: function(bin) {
// Network detection logic
if (/^4/.test(bin)) return 'visa';
if (/^5[1-5]/.test(bin)) return 'mastercard';
if (/^3[47]/.test(bin)) return 'amex';
return 'unknown';
}
};
Security Considerations
-
Data Protection
- Never store full card numbers
- Encrypt BIN data in transit
- Limit BIN data access
-
Compliance Requirements
- PCI DSS compliance for BIN storage
- Data retention policies
- Access control implementation
-
Performance Optimization
- Cache BIN lookup results
- Implement rate limiting
- Use bulk BIN lookups
Best Practices for BIN Processing
Practice | Purpose | Implementation |
---|---|---|
Caching | Reduce API calls | Store BIN data in memory |
Validation | Prevent errors | Check format before processing |
Monitoring | Detect issues | Log BIN lookup patterns |
This guide provides essential information for working with BIN numbers in payment processing systems. Use it to improve payment validation, fraud detection, and transaction routing in your applications.