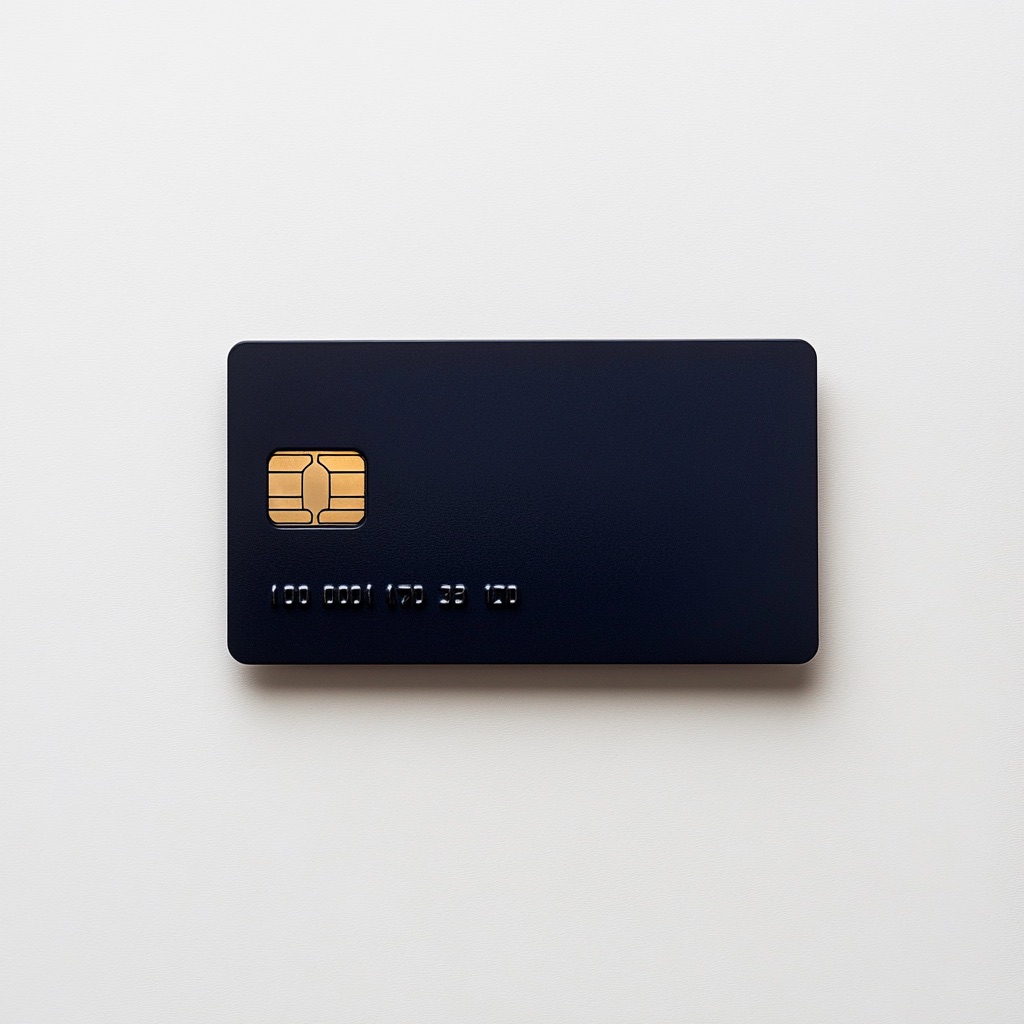
How to Test Credit Card Numbers
Understanding Test Card Numbers
Test credit card numbers are specifically designed numbers that follow the same format and validation rules as real credit cards but cannot process actual payments. These numbers are essential for developers testing payment systems, e-commerce platforms, and financial applications.
Card Number Structure
A credit card number consists of the following components:
Component | Length | Description |
---|---|---|
IIN/BIN | 6 digits | Industry Identifier Number/Bank Identification Number |
Account Number | 6-12 digits | Individual account identifier |
Check Digit | 1 digit | Validates card number using Luhn algorithm |
Common Test Card Numbers by Provider
Visa Test Cards
4532 0151 2345 6789 - Standard Visa
4916 7111 1111 1111 - Visa Debit
4539 9111 1111 1111 - Visa Credit
4485 2755 2489 7845 - Visa Rewards
Mastercard Test Cards
5555 5555 5555 4444 - Standard Mastercard
5105 1051 0510 5100 - Debit Mastercard
5431 1111 1111 1111 - World Mastercard
5200 8282 8282 8210 - Prepaid Mastercard
Response Code Testing Matrix
Card Number End | Response | Use Case |
---|---|---|
...0000 | Approved | Successful transaction |
...0051 | Insufficient funds | Failed payment testing |
...0014 | Invalid card | Error handling testing |
...0098 | Security violation | 3D Secure testing |
Testing Scenarios and Card Numbers
Amount-Based Testing
- Use amount $0.01 - $0.99 for micro-transaction testing
- Use amount $2000+ for high-value transaction testing
- Use amount $99999.99 for limit testing
Special Condition Cards
Last 4 Digits | Test Condition | Expected Response |
---|---|---|
1111 | 3D Secure Required | Authentication redirect |
2222 | Card Verification Failed | CVV error |
3333 | Address Verification Failed | AVS mismatch |
4444 | Rate Limit Testing | Gateway timeout |
Implementation Example
def validate_test_card(card_number):
# Remove spaces and dashes
card = ''.join(filter(str.isdigit, card_number))
# Check length (13-19 digits)
if not (13 <= len(card) <= 19):
return False
# Luhn algorithm check
sum = 0
is_even = False
for digit in reversed(card):
digit = int(digit)
if is_even:
digit *= 2
if digit > 9:
digit -= 9
sum += digit
is_even = not is_even
return sum % 10 == 0
Best Practices for Test Card Usage
-
Environment Isolation
- Never use test cards in production
- Maintain separate test and production API keys
- Use different endpoints for test/production
-
Data Security
- Don't log full test card numbers
- Mask card numbers in logs (e.g., ************1234)
- Rotate test cards periodically
-
Testing Coverage
- Test all card types your system accepts
- Include declined transaction scenarios
- Test card expiry and CVV validation
Troubleshooting Common Issues
Issue | Solution | Prevention |
---|---|---|
Invalid card error | Verify Luhn check | Implement client-side validation |
Gateway timeout | Check API endpoints | Add retry logic |
Authentication failure | Verify API keys | Use environment variables |
Additional Resources
This guide provides the essential information for implementing and testing credit card processing in your development environment. Remember to always use test card numbers in development and never attempt to process real credit card numbers in test environments.